Tutorial: Build your own custom field plugin - tmpl/owl.php
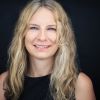
Written by Elisa Foltyn
tmpl/owl.php
Now we create the output of our template.
<?php
/**
* @package Joomla.Plugin
* @subpackage Fields.Owlimg
*
* @copyright Copyright (C) 2005 - 2016 Open Source Matters, Inc. All rights reserved.
* @license GNU General Public License version 2 or later; see LICENSE.txt
*/
defined('_JEXEC') or die;
JLoader::register('JFolder', JPATH_LIBRARIES . '/joomla/filesystem/folder.php');
$folder = $field->value;
if (!$folder)
{
return;
}
With the class JLoader:register
and the Method JFolder we import the library folder.php
.
We assign the field value to our variable $folder
. If the field value is empty, the output is empty.
Now we call some parameters and assign them to our variables.
$items = $fieldParams->get('items', '3');
(See the file in the download package for a full view)
The first value inside the brackets is the name of the parameter, the second value is the default value if no parameter value is provided.
$images = JFolder::files('images/' . $folder);
if (!empty($images))
{
$folderpath = 'images/' . $folder . '/';
How do we now retrieve our image path? With the class JFolder::files
we retrieve the file names inside a Joomla! directory. Therefore, we collect in the $images
variable the paths to all images in the selected folder. Afterwards, we wrap the code in an if query to make sure, that the code is only executed if the folder is not empty.
JHtml::_('jquery.framework');
JHtml::_('script', 'media/plg_fields_owlimg/js/owl.carousel.min.js');
JHtml::_('stylesheet', 'media/plg_fields_owlimg/css/owl.carousel.min.css');
JHtml::_('stylesheet', 'media/plg_fields_owlimg/css/owl.theme.default.min.css');
if ($animatein || $animateout)
{
JHtml::_('stylesheet', 'media/plg_fields_owlimg/css/animate.min.css');
}
?>
With JHtml::_('jquery.framework');
we call the jQuery framework and make sure it's called BEFORE any other of our scripts. That's important for it to function correctly.
Next, we load our script and stylesheet files. By using the JHtml::_
class we enable template developers to override our files in a template override. We use the methods script
and stylesheet
.
The Advantage: The template developer can create a file named yourtemplate/html/css/plg_fields_owlimg/owl.carousel.min.css
and Joomla! automatically recognises that the file media/plg_fields_owlimg/css/owl.carousel.min.css
has to be overridden.
<div class="owl-carousel <?php echo ($customclass) ? $customclass : ''; ?> owl-theme">
<?php foreach ($images as $image) : ?>
<div class="item">
<img src="/%3C?php_echo_%24folderpath___%24image%3B_%3F%3E=">
</div>
<?php endforeach; ?>
</div>
Now we create a container for the owl carousel and import a unique class name from the parameters. If no class name is set, we pull the default value "myowl".
Note: If more "owls" are shown on one page, the class name has to be unique.
<script>
(function ($) {
$('.<?php echo ($customclass) ? $customclass : ''; ?>').owlCarousel({
<?php echo ($items) ? 'items:' . $items . ',' : ''; ?>
<?php echo ($margin) ? 'margin:' . $margin . ',' : ''; ?>
<?php echo ($stagepadding) ? 'stagePadding:' . $stagepadding . ',' : ''; ?>
<?php echo ($center) ? 'center:' . $center . ',' : ''; ?>
<?php echo ($autowidth) ? 'autoWidth:' . $autowidth . ',' : ''; ?>
<?php echo ($loop) ? 'loop:' . $loop . ',' : ''; ?>
<?php echo ($rewind) ? 'rewind:' . $rewind . ',' : ''; ?>
<?php echo ($lazyload) ? 'lazyLoad:' . $lazyload . ',' : ''; ?>
<?php echo ($mousedrag) ? 'mouseDrag:' . $mousedrag . ',' : ''; ?>
<?php echo ($touchdrag) ? 'touchDrag:' . $touchdrag . ',' : ''; ?>
<?php echo ($pulldrag) ? 'pullDrag:' . $pulldrag . ',' : ''; ?>
<?php echo ($freedrag) ? 'freeDrag:' . $freedrag . ',' : ''; ?>
<?php echo ($nav) ? 'nav:' . $nav . ',' : ''; ?>
<?php echo ($nav && $navtext) ? 'navText:[' . $navtext . '],' : ''; ?>
<?php echo ($dots) ? 'dots:' . $dots . ',' : ''; ?>
<?php echo ($autoplay) ? 'autoplay:' . $autoplay . ',' : ''; ?>
<?php echo ($autoplay && $hoverpause) ? 'autoplayHoverPause:' . $hoverpause . ',' : ''; ?>
<?php echo ($autoplay && $autoplayspeed) ? 'autoplaySpeed:' . $autoplayspeed . ',' : ''; ?>
<?php echo ($animatein) ? "animateIn:'" . $animatein . "'," : ""; ?>
<?php echo ($animateout) ? "animateOut:'" . $animateout . "'," : ""; ?>
<?php echo ($responsive) ? $responsiveoptions : ''; ?>
});
})(jQuery);
</script>
<?php
}
In the end, we initiate the owl carousel and include the variables that pull the parameter values.